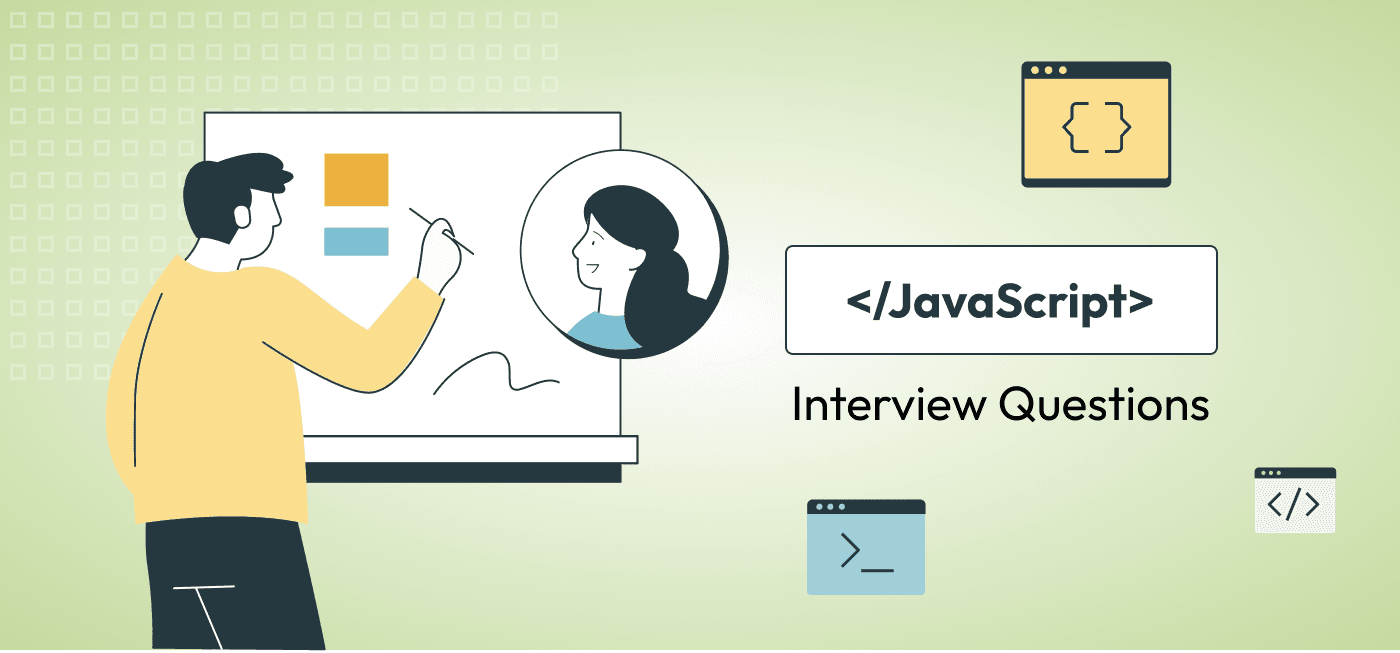
Hiring + recruiting | Blog Post
15 JavaScript Interview Questions for Hiring JavaScript Engineers
Todd Adams
Share this post
In the dynamic landscape of web development, JavaScript is a critical skill for creating interactive and responsive web applications. The demand for proficient JavaScript engineers is consistently high, reflecting the language’s central role in web technology. This list of JavaScript interview questions is tailored to assist hiring managers and technical recruiters in identifying candidates with the necessary expertise in JavaScript. These JavaScript interview questions span a broad range of topics, from fundamental concepts to complex functionalities and best practices, providing a comprehensive overview of a candidate’s proficiency in JavaScript.
JavaScript Interview Questions
Q1: What are the differences between var
, let
, and const
in JavaScript?
Question Explanation
This question probes the candidate’s understanding of variable scopes, declaration keywords, and their peculiarities in JavaScript—a foundational concept that affects code structure, error prevention, and memory management.
Expected Answer
- Scope:
var
declarations are function-scoped or globally scoped if declared outside a function, which means they are accessible throughout the function. In contrast,let
andconst
are block-scoped, making them only accessible within the nearest enclosing block (like anif
statement or a loop). - Hoisting: Variables declared with
var
are hoisted to the top of their function or global scope and are initialized withundefined
, meaning they can be used before their declaration.let
andconst
are also hoisted but not initialized, creating a temporal dead zone from the block start to the declaration line. - Reassignment and Re-declaration: Variables declared with
var
can be re-declared and updated within its scope.let
allows updating but not re-declaration in the same scope, offering a safer option to prevent accidental variable re-creation.const
is for constants; it cannot be updated or re-declared once initialized, ensuring the variable’s value remains the same throughout the script.
Evaluating Responses
Look for responses that accurately describe the differences in scope, hoisting, and the mutability of these variable declarations. A good answer may also include examples to illustrate these concepts and might touch upon specific use cases or pitfalls associated with each declaration type.
Q2: Can you explain how JavaScript’s event loop works?
Question Explanation
Understanding the event loop is essential for JavaScript developers, especially given the language’s single-threaded nature. This question tests the candidate’s grasp of asynchronous programming concepts in JavaScript, including how operations are handled outside the main program flow.
Expected Answer
- Event Loop Basics: JavaScript has a single-threaded, non-blocking event loop mechanism. The event loop allows JavaScript to perform non-blocking asynchronous operations by using a call stack, an event queue, and a heap. The call stack executes synchronous code, the heap stores memory allocation, and the event queue holds asynchronous callbacks.
- Runtime Behavior: When the call stack is empty, the event loop checks the event queue. If there’s any waiting callback, it’s dequeued and pushed onto the call stack to be executed. This cycle allows the event loop to perform long-running tasks (like I/O operations) asynchronously, without blocking the main thread.
- Microtasks and Macrotasks: JavaScript further categorizes tasks into microtasks (e.g., promises) and macrotasks (e.g.,
setTimeout
,setInterval
). Microtasks have higher priority and are executed before the next macrotask begins, ensuring that asynchronous operations like promise resolution happen as soon as possible.
Evaluating Responses
Candidates should clearly articulate how the event loop works, distinguishing between the call stack, event queue, and heap. A comprehensive answer will include the distinction between microtasks and macrotasks, demonstrating a nuanced understanding of JavaScript’s execution model and its implications for asynchronous programming.
Q3: How do you handle errors and exceptions in JavaScript?
Question Explanation
Error handling is a critical part of programming, ensuring that applications can gracefully recover from unexpected situations. This question assesses the candidate’s approach to identifying, managing, and mitigating errors in JavaScript applications.
Expected Answer
- try…catch Statement: The primary mechanism for catching exceptions in JavaScript is the
try...catch
statement. Code that might throw an error is placed in thetry
block. If an error occurs, execution moves to thecatch
block, allowing the error to be handled gracefully. - finally Block: Optionally, a
finally
block can be used, which executes after the try and catch blocks, regardless of whether an exception was caught. It’s useful for cleaning up resources, like closing file streams or database connections. - Error Objects: When an error is caught, it can be accessed as an object with properties like
name
,message
, andstack
. This allows for more detailed error reporting and handling based on different error types.
Example:
try {
// Code that may throw an error
throw new Error('This is an error message');
} catch (error) {
console.error(`Error caught: ${error.message}`);
} finally {
console.log('This will run regardless of the try / catch outcome');
}
Evaluating Responses
The response should cover the use of try...catch
for catching exceptions, the optional finally
block for cleanup, and an understanding of error objects for detailed error information. Candidates who provide examples or discuss error handling strategies, including custom error types or logging practices, demonstrate a deeper understanding of robust JavaScript application development.
Q4: Describe the concept and uses of closures in JavaScript.
Question Explanation
Closures are a fundamental and powerful feature of JavaScript, enabling function encapsulation and the creation of private variables. This JavaScript interview question tests the candidate’s understanding of scope, closures, and how they can be used to maintain state in a function after its execution context has ended.
Expected Answer
- Basic Concept: A closure is created when a function is defined within another function, allowing the inner function to access and manipulate variables from the outer function’s scope, even after the outer function has finished execution. This behavior is possible because functions in JavaScript form closures around the data they can access.
- Uses: Closures are used for data encapsulation and creating factory and module patterns, where private variables and functions can be shielded from the global scope, thus preventing potential name clashes and ensuring data integrity. They’re also essential in callback functions, event handlers, and functions that work with external data, like API calls, where they maintain state between asynchronous operations.
Example:
function createCounter() {
let count = 0; // `count` is a private variable created by the closure
return function() {
count += 1; // Access and modify `count` within the closure
return count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
Evaluating Responses
Candidates should clearly explain what closures are, how they are created, and their practical uses, particularly in creating private variables and maintaining state. Look for answers that include examples demonstrating a closure’s ability to encapsulate data and the reasoning behind using them in specific scenarios.
Q5: Explain the importance and functionality of prototypes in JavaScript.
Question Explanation
Prototypes are a core concept in JavaScript, pivotal for understanding object-oriented programming in the language. This question assesses the candidate’s knowledge of prototypal inheritance and its role in object creation and behavior delegation.
Expected Answer
- Basic Concept: Every JavaScript object has a
prototype
property, which is a reference to another object. Thisprototype
object contains common properties and methods that are accessible to the object, enabling prototypal inheritance. This means objects can inherit properties and methods from their prototype. - Importance: Prototypes are essential for defining methods and properties that should be shared across instances of an object or constructor function. They enable efficient memory usage, as shared methods are stored on the prototype, rather than duplicated in each instance.
Example:
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log('Hello, my name is ' + this.name);
};
const john = new Person('John');
john.greet(); // Outputs: Hello, my name is John
Evaluating Responses
A well-rounded response should cover the concept of prototypes, their role in prototypal inheritance, and their practical uses in JavaScript. Examples that demonstrate how prototypes allow for property and method sharing across instances can showcase the candidate’s practical knowledge of prototypes in JavaScript.
Q6: How do promises improve asynchronous programming in JavaScript?
Question Explanation
Promises represent a significant advancement in handling asynchronous operations in JavaScript, providing a more manageable and readable approach than traditional callback functions. This JavaScript interview question evaluates the candidate’s understanding of asynchronous programming patterns and their experience with Promises.
Expected Answer
- Basic Concept: A Promise in JavaScript is an object representing the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises allow for asynchronous operations to be handled using a more straightforward and cleaner syntax, improving code readability and error handling.
- Improvements Over Callbacks: Promises provide a way to avoid callback hell, offering a chainable solution where operations can be sequenced and errors can be caught and handled effectively. They allow for the execution of asynchronous code in a manner that appears more synchronous and linear.
Example:
function getData(url) {
return new Promise((resolve, reject) => {
// Simulate an asynchronous operation
setTimeout(() => {
const data = 'Sample data';
resolve(data);
}, 1000);
});
}
getData('https://api.example.com/data')
.then(data => console.log(data)) // Process data
.catch(error => console.error(error)); // Handle errors
Evaluating Responses
Candidates should describe promises as a tool for improving the handling of asynchronous operations, mentioning their advantages over callbacks, such as better error handling and the ability to chain operations. Examples should illustrate how promises are used to manage asynchronous code flow more cleanly and efficiently.
Q7: What are arrow functions, and how do they differ from traditional functions?
Question Explanation
Arrow functions, introduced in ES6, offer a more concise syntax for writing function expressions in JavaScript. This question tests the candidate’s familiarity with ES6+ syntax and the functional differences between arrow functions and traditional function expressions.
Expected Answer
- Syntax and Conciseness: Arrow functions provide a shorter syntax compared to traditional functions. They omit the
function
keyword and use an arrow (=>
) to separate parameters from the function body. For single-expression functions, curly braces and thereturn
statement are optional. this
Binding: Unlike traditional functions, arrow functions do not have their ownthis
context. Instead,this
is lexically bound, meaning it usesthis
from the enclosing execution context. This property makes arrow functions particularly useful for callbacks and methods inside objects where a specificthis
context is required.- Use Cases and Limitations: Arrow functions are ideal for simple operations, especially as arguments to higher-order functions. However, they cannot be used as constructors, and they do not have their own
arguments
object.
Example:
const traditionalFunc = function(a, b) {
return a + b;
};
const arrowFunc = (a, b) => a + b;
// Traditional function used as a method
const obj = {
value: 1,
increment: function() { this.value += 1; }
};
// Arrow function used as a method (not recommended for this case)
const objWithArrow = {
value: 1,
increment: () => this.value += 1 // `this` does not bind to objWithArrow, but to the outer context
};
Evaluating Responses
Good answers should highlight the syntactical differences between arrow functions and traditional functions, the lexically scoped this
behavior, and appropriate use cases for arrow functions. Awareness of limitations, like the inability to use arrow functions as constructors, reflects a deeper understanding of function types in JavaScript.
Q8: Can you describe the process of event delegation in JavaScript?
Question Explanation
Event delegation is a technique used in JavaScript to handle events efficiently, especially in scenarios involving multiple similar elements. This concept is essential for understanding event handling, bubbling, and the efficient management of dynamic content in web applications.
Expected Answer
- Basic Concept: Event delegation leverages the event bubbling phase, where events propagate from the target element up through the ancestors in the DOM tree. Instead of attaching an event listener to each individual element, event delegation attaches a single event listener to a parent element. This listener reacts to events coming from its child elements, determining the target element through event properties.
- Benefits: This approach reduces the memory footprint by minimizing the number of event listeners and simplifies the management of event listeners for dynamically added or removed elements. It is particularly useful in handling events on elements that do not exist at the time the page loads but may be added dynamically.
Example:
document.getElementById('parentElement').addEventListener('click', function(event) {
if (event.target.tagName === 'LI') { // Checks if the target of the click is an LI element
console.log('List item ', event.target.id, ' was clicked.');
}
});
Evaluating Responses
Candidates should clearly explain the mechanism of event delegation, its advantages, and provide a straightforward example demonstrating how it works. Understanding event delegation indicates proficiency in managing events and optimizing web application performance.
Q9: What is the significance of the this
keyword in JavaScript?
Question Explanation
The this
keyword is a fundamental concept in JavaScript and a useful JavaScript interview question, associated with execution contexts and object-oriented programming. Understanding this
is crucial for working with object methods and functions, especially when dealing with event handlers and callbacks.
Expected Answer
- Basic Concept: In JavaScript,
this
refers to the object that is executing the current function. The value ofthis
depends on how the function is called, not where it is defined. - Contexts:
- In a method,
this
refers to the owner object. - In a regular function,
this
refers to the global object (window
in a browser,global
in Node.js), orundefined
in strict mode. - For functions defined as arrow functions,
this
is lexically scoped, meaning it usesthis
from the enclosing execution context.
- In a method,
- Binding
this
: The value ofthis
can be explicitly set with methods likecall()
,apply()
, andbind()
.
Example:
const person = {
name: 'John',
greet: function() { console.log('Hi, my name is ' + this.name); }
};
person.greet(); // Hi, my name is John
const greet = person.greet;
greet(); // Hi, my name is undefined (or a global object name in non-strict mode)
const greetBound = greet.bind(person);
greetBound(); // Hi, my name is John
Evaluating Responses
Look for explanations that clearly articulate different scenarios where this
might vary and highlight the importance of understanding this
for managing execution context. Examples should demonstrate the candidate’s ability to manipulate and correctly predict the value of this
in various contexts.
Q10: How can you achieve inheritance in JavaScript?
Question Explanation
Inheritance is a key concept in object-oriented programming, allowing objects to inherit properties and methods from other objects. This question examines the candidate’s understanding of JavaScript’s prototypal inheritance model and their ability to apply it to create hierarchical object structures.
Expected Answer
- Prototypal Inheritance: JavaScript implements inheritance through prototypes. Each object has a property called
prototype
, where you can add methods and properties that other objects can inherit. TheObject.create()
method can be used to create a new object with the specified prototype object and properties. - Constructor Functions and Class Syntax: Before ES6, constructor functions were used to simulate a class-like inheritance structure. ES6 introduced the
class
syntax, making inheritance easier to implement and understand. Theextends
keyword creates a class as a child of another class.
Example:
Using constructor functions:
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a noise.');
};
function Dog(name) {
Animal.call(this, name); // Super call
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.speak = function() {
console.log(this.name + ' barks.');
};
var dog = new Dog('Rex');
dog.speak(); // Rex barks.
Using class syntax:
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(this.name + ' makes a noise.');
}
}
class Dog extends Animal {
speak() {
console.log(this.name + ' barks.');
}
}
const dog = new Dog('Rex');
dog.speak(); // Rex barks.
Evaluating Responses
Effective answers will discuss both prototypal inheritance and the use of constructor functions or class syntax to achieve inheritance in JavaScript. The inclusion of examples showcasing both methods provides a comprehensive understanding of JavaScript’s inheritance mechanisms.
Q11: Explain the concept of hoisting in JavaScript.
Question Explanation
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope before code execution. Understanding hoisting is crucial for debugging unexpected behaviors and writing predictable JavaScript code.
Expected Answer
- Basic Concept: In JavaScript, before the execution of code, all variable declarations (declared with
var
) and function declarations are hoisted to the top of their scope. This means they are processed before any code is executed. However, the assignments are not hoisted. var
,let
, andconst
: Variables declared withvar
are hoisted and initialized withundefined
. Variables declared withlet
andconst
are also hoisted but remain uninitialized, forming a temporal dead zone until their declaration is executed in the code.- Function Declarations and Expressions: Function declarations are hoisted, so they can be called before they are defined in the code. Function expressions, including those defined with arrow functions, are treated according to the variable declaration (
var
,let
,const
) and follow the respective hoisting rules.
Example:
console.log(x); // undefined
var x = 5;
console.log(y); // ReferenceError: Cannot access 'y' before initialization
let y = 10;
hoistedFunction(); // Outputs: "This function has been hoisted."
function hoistedFunction() {
console.log("This function has been hoisted.");
};
nonHoistedFunction(); // TypeError: nonHoistedFunction is not a function
var nonHoistedFunction = function() {
console.log("This function expression is not hoisted.");
};
Evaluating Responses
A comprehensive answer will explain the mechanism of hoisting and its effects on different types of declarations in JavaScript. Examples illustrating the concept, especially the differences in behavior between var
, let
, and const
, and between function declarations and expressions, demonstrate a
Q12: What are template literals, and how do they enhance string manipulation?
Question Explanation
Template literals, introduced in ES6, provide a more powerful and convenient syntax for creating complex strings. This question assesses the candidate’s familiarity with modern JavaScript features and their ability to use them for effective string manipulation and dynamic content generation.
Expected Answer
- Basic Concept: Template literals are enclosed by backticks (
`
) instead of single or double quotes. They allow for embedding expressions within strings, which can be variables, operations, or function calls, evaluated and converted into a resulting string. - Enhancements Over Traditional Strings:
- Multi-line strings: Easily create strings that span multiple lines without needing to use newline characters explicitly.
- Interpolation: Embed expressions directly within the string using
${expression}
, simplifying concatenation and dynamic content creation. - Tagged Templates: Extend their functionality with tagged templates, allowing the parsing of template literals with a function. The tag function can manipulate the output of the template literal, providing a way to customize the string processing.
Example:
const name = 'World';
console.log(`Hello, ${name}!`); // Outputs: Hello, World!
// Multi-line string
const greeting = `Hello,
${name}!`;
console.log(greeting);
// Tagged template literal
function tag(strings, value) {
return `${strings[0]}${value.toUpperCase()}!`;
}
console.log(tag`Hello, ${name}`); // Outputs: Hello, WORLD!
Evaluating Responses
Candidates should mention the syntax of template literals, their benefits for creating multi-line strings and embedding expressions, and the concept of tagged templates. Examples demonstrating these features show practical understanding and the ability to leverage template literals for more readable and concise code.
Q13: Discuss the methods for deep cloning an object in JavaScript.
Q13: Discuss the methods for deep cloning an object in JavaScript.
Question Explanation
Deep cloning objects is a common necessity in JavaScript, especially to avoid unintended side effects due to the mutable nature of objects. This question evaluates the candidate’s knowledge of creating entirely independent copies of objects, including nested structures.
Expected Answer
- JSON Method: One common but limited method involves serializing an object to a JSON string and then parsing it back to a new object. This works for simple cases but does not handle functions,
undefined
, or circular references. - Structured Clone Algorithm: A more robust method introduced in recent JavaScript environments is the
structuredClone()
function, which can clone a wider variety of types, including Maps, Sets, Dates, regular expressions, and more, handling circular references as well. - Manual Cloning: Manually implementing a deep clone function using recursion to copy each property and check for nested objects. This method is customizable and can handle specific requirements but is more complex to implement correctly.
- Libraries: Utilizing libraries like Lodash, which offer deep cloning functions (
_.cloneDeep
) that are well-tested and can handle various edge cases.
Example:
Using structuredClone()
:
const original = { name: 'John', meta: { age: 30, city: 'New York' }};
const clone = structuredClone(original);
Using Lodash:
const clone = _.cloneDeep(original);
Evaluating Responses
Effective answers will cover multiple methods for deep cloning, including their advantages and limitations. Candidates should be aware of the JSON method’s limitations and the more versatile structuredClone()
function. Demonstrating knowledge of manual deep cloning techniques or the use of libraries indicates a comprehensive understanding of handling complex object cloning.
Q14: What are higher-order functions, and provide examples of their use?
Question Explanation
Higher-order functions are a cornerstone of functional programming in JavaScript, enabling powerful patterns and techniques. This question tests the candidate’s understanding of functional programming concepts and their ability to leverage these functions in JavaScript.
Expected Answer
- Basic Concept: Higher-order functions are functions that can take other functions as arguments or return a function as their result. This allows for abstracting or encapsulating behaviors, making code more modular, reusable, and expressive.
- Examples:
- Array Methods:
.map()
,.filter()
,.reduce()
are native JavaScript higher-order functions that operate on arrays, taking a callback function to apply operations on each element. - Custom Higher-Order Functions: Creating functions that configure and return other functions, often used in creating configurable middleware or components in libraries and frameworks.
- Array Methods:
Example:
Using .map()
:
const numbers = [1, 2, 3, 4];
const squaredNumbers = numbers.map(number => number * number);
console.log(squaredNumbers); // Outputs: [1, 4, 9, 16]
Custom higher-order function:
function greaterThan(n) {
return m => m > n;
}
let greaterThan10 = greaterThan(10);
console.log(greaterThan10(11)); // true
Evaluating Responses
Candidates should explain the concept of higher-order functions and their utility in JavaScript programming, providing examples of both built-in higher-order functions and scenarios where custom higher-order functions are useful. Good responses will demonstrate how these functions contribute to more expressive and flexible code.
Q15: How does JavaScript’s asynchronous and non-blocking nature affect performance?
Question Explanation
JavaScript’s ability to perform asynchronous operations and non-blocking I/O is pivotal for creating responsive applications. This JavaScript interview question probes the candidate’s understanding of JavaScript’s execution model and its impact on application performance and user experience.
Expected Answer
- Asynchronous Operations: JavaScript’s event loop and callback queue allow it to perform long-running tasks (like network requests or file operations) without blocking the main thread. This ensures that the user interface remains responsive while waiting for these tasks to complete.
- Non-blocking I/O: Utilizing non-blocking I/O operations (such as those in Node.js) enables efficient handling of concurrent operations, making it suitable for high-performance, scalable applications that require handling multiple requests simultaneously.
- Promises and Async/Await: The introduction of Promises and async/await syntax has further improved handling asynchronous operations, allowing for more readable and manageable code, which can lead to better error handling and potentially fewer performance bottlenecks.
- Impact on Performance: Properly leveraging JavaScript’s asynchronous nature can significantly enhance application performance and responsiveness. However, mismanagement (like excessive callbacks or poorly structured Promise chains) can lead to issues such as callback hell, memory leaks, or event loop blockage, adversely affecting performance.
Evaluating Responses
A comprehensive answer should discuss the benefits of asynchronous programming and non-blocking I/O in JavaScript, the evolution of practices with Promises and async/await, and the potential performance implications. Candidates who acknowledge the importance of understanding and effectively managing asynchronous operations demonstrate an awareness of the challenges and best practices in developing high-performance JavaScript applications.
Conclusion
These 15 JavaScript interview questions have been curated to delve into a candidate’s understanding, experience, and approach to solving common and advanced problems using JavaScript. The aim is to evaluate not just the technical knowledge but also the practical application and problem-solving skills in real-world scenarios. Whether it’s handling asynchronous operations, managing scope, or understanding the core principles that underpin JavaScript, a candidate’s responses to these questions can offer deep insights into their capabilities and fit for a role as a JavaScript engineer. Hiring individuals who not only understand the syntax but also the nuances and best practices of JavaScript is key to building robust, efficient, and scalable web applications.