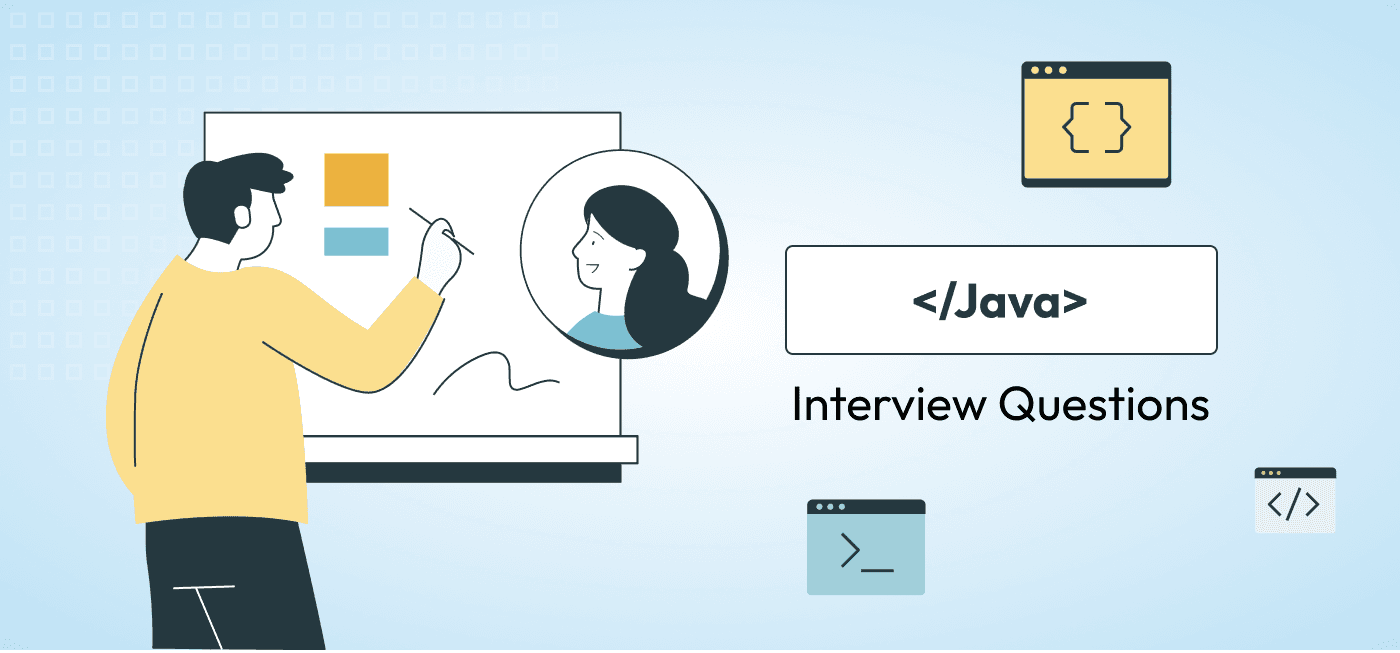
Hiring + recruiting | Blog Post
15 Java Interview Questions and Answers for Hiring Java Engineers
Todd Adams
Share this post
This post aims to provide a comprehensive set of interview questions tailored specifically for the recruitment of proficient Java engineers. In the rapidly evolving field of software development, Java remains a cornerstone language, known for its versatility, reliability, and widespread use in everything from web applications to enterprise software. The following Java interview questions and answers are designed to probe the depth and breadth of a candidate’s knowledge in Java, assessing not just theoretical understanding but practical application and problem-solving skills as well.
Java Interview Questions
Q1: Explain the differences between JDK, JRE, and JVM.
Question Explanation:
Understanding the differences between JDK, JRE, and JVM is fundamental for any Java developer, as it lays the groundwork for how Java applications are developed, run, and managed. These components are interrelated, each serving a unique role in the lifecycle of a Java application.
Expected Answer:
- JVM (Java Virtual Machine): JVM is an abstract machine that provides the runtime environment for Java bytecodes to be executed. It is platform-dependent, meaning there’s a specific JVM for each operating system, which allows Java to be platform-independent. JVM is responsible for loading code, verifying code, executing code, and providing runtime environment.
- JRE (Java Runtime Environment): JRE comprises the JVM and the core libraries and components needed for running Java applications. It does not include development tools such as compilers or debuggers. Essentially, JRE is the on-disk system that takes your Java code, combines it with the necessary libraries, and starts the JVM to execute it.
- JDK (Java Development Kit): JDK is a superset of JRE and includes everything within the JRE plus tools such as the compilers (
javac
), debuggers, and documentation tools. It is the complete package for developing, compiling, and running Java applications.
Evaluating Responses:
Candidates should demonstrate a clear understanding of the roles and responsibilities of JVM, JRE, and JDK. They should articulate that JVM is the platform-dependent engine that runs the bytecode, JRE is the container that provides the runtime environment, and JDK is the complete development kit for building and running Java applications. Bonus points for examples or analogies that help clarify their explanations.
Q2: How does Java achieve platform independence?
Question Explanation:
Java’s platform independence is a cornerstone feature that allows developers to write applications once and run them on any device equipped with a JVM. This Java interview question probes the candidate’s understanding of Java’s compile-time and runtime processes.
Expected Answer:
Java achieves platform independence through its compilation and runtime process. Java programs are compiled into bytecode, which is a platform-independent code, by the Java compiler (javac). This bytecode is not executed directly by the hardware but by the JVM, which interprets or compiles the bytecode into native machine code at runtime. Since JVMs are available for many different operating systems, the same Java bytecode can be run on any platform that has a compatible JVM, making the program platform-independent.
Evaluating Responses:
Look for explanations that emphasize the role of bytecode and the JVM. A strong answer will describe the process of compiling Java source code into bytecode and the role of JVM in interpreting this bytecode for the native machine. Understanding the distinction between compile-time activities and runtime execution is key.
Q3: What is the significance of the final
keyword in Java?
Question Explanation:
The final
keyword in Java is a modifier that denotes immutability and finality in different contexts. This question tests the candidate’s understanding of Java’s approach to security, design, and optimization.
Expected Answer:
The final
keyword in Java can be applied to variables, methods, and classes:
- Variables: A final variable cannot change its value once initialized. It can be set either in its declaration or within the constructor. For primitives, this means the value is constant. For object references, it means the reference cannot be changed, though the object itself can be mutable.
- Methods: A final method cannot be overridden by subclasses. This is useful for security, to prevent alteration of behavior, and for design, to ensure consistent behavior across subclasses.
- Classes: A final class cannot be subclassed. This is particularly useful when creating immutable classes or securing functionality that should not be altered.
Evaluating Responses:
Candidates should accurately describe the use of final
for variables, methods, and classes, highlighting its role in ensuring immutability, preventing method overriding, and blocking class inheritance. Responses should reflect an understanding of how final
contributes to Java’s security and design principles.
Q4: Can you explain the concept of the Singleton pattern in Java? Provide an example.
Question Explanation:
The Singleton pattern is a fundamental design pattern that ensures a class has only one instance and provides a global point of access to it. This question assesses the candidate’s grasp of design patterns and their implementation in Java.
Expected Answer:
The Singleton pattern is implemented in Java by making the constructor private, defining a static variable that holds the instance, and providing a static method that returns this instance. Lazy initialization and thread safety are common considerations in its implementation.
Example:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
This example demonstrates a thread-safe, lazily initialized Singleton. The instance
is only created when getInstance()
is called, ensuring that the class is loaded only when necessary. The synchronized block within the null check provides thread safety.
Evaluating Responses:
Look for a clear explanation of the Singleton pattern, including its purpose (to ensure a single instance of a class) and key components (private constructor, static instance variable, static factory method). The example should correctly demonstrate a way to implement a Singleton in Java, with bonus points for addressing lazy initialization and thread safety issues.
Q5: How does Java handle memory management?
Q5: How does Java handle memory management?
Question Explanation:
Java’s memory management involves automatic garbage collection, which helps in managing application memory by freeing up memory used by objects that are no longer in use. This question evaluates a candidate’s understanding of Java’s approach to memory management and its impact on application performance.
Expected Answer:
Java uses a garbage collector to automate memory management. Memory in Java is divided into two main areas: heap and stack. The heap is where Java objects live, and the stack is where methods and local variables are stored. The garbage collector works primarily on the heap.
- Garbage Collection: Java’s garbage collector periodically checks for objects that are no longer referenced by any part of the program. These objects are considered garbage and are removed to free up memory. The JVM uses various garbage collection algorithms, including “Mark and Sweep,” “Generational Collection,” and others, to efficiently manage memory.
- Heap Management: The heap is divided into generations (young, old, and permanent generations) to optimize garbage collection performance. Most objects are created in the young generation and moved to the older generation if they live long enough.
- Stack Management: Each thread in a Java application has its own stack, which stores primitive variables and references to objects in the heap. The stack is cleaned up automatically as methods finish execution.
Evaluating Responses:
Candidates should demonstrate an understanding of garbage collection and its role in memory management, including the concepts of heap and stack. They should be able to explain how garbage collection works, its impact on application performance, and any strategies for optimizing memory usage in Java applications.
Q6: Describe the differences between checked and unchecked exceptions in Java.
Question Explanation:
Java’s exception handling framework distinguishes between checked and unchecked exceptions. This distinction affects how a developer handles error conditions in code. This question assesses the candidate’s knowledge of Java’s error handling mechanism.
Expected Answer:
- Checked Exceptions: Checked exceptions are exceptions that must be either caught or declared in the method signature. These are conditions that a well-written application should anticipate and recover from. Examples include
IOException
andSQLException
. Checked exceptions are subject to compile-time checking, ensuring that these errors are handled throughtry-catch
blocks or declared with thethrows
keyword. - Unchecked Exceptions: Unchecked exceptions include runtime exceptions (subclasses of
RuntimeException
) and errors (subclasses ofError
). These exceptions are not subject to compile-time checking, and it’s up to the developer to use discretion in handling these. Unchecked exceptions usually indicate programming errors, such as bugs or incorrect use of an API. Examples includeNullPointerException
,ArrayIndexOutOfBoundsException
, andStackOverflowError
.
Evaluating Responses:
Responses should clearly distinguish between checked and unchecked exceptions, including their definition, examples, and how they are handled or declared in code. A strong answer might also touch on the debate over the use of checked versus unchecked exceptions and best practices for error handling in Java.
Q7: What is the purpose of the static
keyword in Java? Give examples of its use.
Question Explanation:
The static
keyword in Java is used to indicate that a particular field, method, or block belongs to the class, rather than instances of the class. This question probes the candidate’s understanding of class-level features and how they’re used in Java programming.
Expected Answer:
The static
keyword serves several purposes:
- Static Variables: These are class-level variables shared by all instances of the class. For example, if you declare a static variable
counter
in a class, all instances of that class share the samecounter
. Static variables are often used to keep count of the number of instances of a class. - Static Methods: These are methods that can be called without creating an instance of the class. They only have access to static variables and other static methods. Example:
Math.sqrt()
, wheresqrt
is a static method of theMath
class. - Static Blocks: Static initialization blocks are executed once when the class is loaded into memory. They are typically used to perform initialization of static variables.
Evaluating Responses:
Look for responses that accurately describe the uses of the static
keyword for variables, methods, and blocks. Candidates should provide clear examples for each and explain why and when the static
keyword might be used in Java programming.
Q8: Explain polymorphism in Java. Can you provide a practical example?
Question Explanation:
Polymorphism is a fundamental concept in object-oriented programming that allows objects to be treated as instances of their parent class rather than their actual class. This question tests the candidate’s understanding of polymorphism and its application in Java.
Expected Answer:
Polymorphism in Java is the ability of an object to take many forms. It allows Java objects to be accessed through references with their own type or references of their superclass types. The two main types of polymorphism in Java are compile-time (or static) polymorphism and runtime (or dynamic) polymorphism.
- Compile-time Polymorphism: Achieved through method overloading. This means multiple methods can have the same name with different parameters within the same class.
- Runtime Polymorphism: Achieved through method overriding, where a subclass has a method with the same name, return type, and parameters as a method in its superclass.
Example:
class Animal {
void sound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Woof");
}
}
public class TestPolymorphism {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // Outputs: Woof
}
}
This example demonstrates runtime polymorphism where an Animal
reference type is used to invoke the sound
method of the Dog
object, resulting in the Dog
class’s sound
method being called.
Evaluating Responses:
Candidates should explain both compile-time and runtime polymorphism, providing clear examples of each. Look for explanations that include the use of method overloading and overriding to achieve polymorphism and practical examples that illustrate these concepts in action.
Q9: What are the benefits of using generics in Java?
Question Explanation:
Generics were introduced to the Java language to provide tighter type checks at compile time and to support generic programming, allowing classes, interfaces, and methods to operate on objects of various types while providing compile-time type safety. This question explores the candidate’s understanding of generics and their advantages in Java programming.
Expected Answer:
The benefits of using generics in Java include:
- Type Safety: Generics enforce type checks at compile time, preventing runtime errors by ensuring that only the correct type of objects are stored in a collection. This makes the code safer and easier to read.
- Elimination of Type Casting: With generics, there’s no need for explicit type casting when retrieving an object from a collection because the compiler already knows the type of elements in the collection.
- Code Reuse: Generics enable classes and methods to be parameterized, meaning they can work with any type of data, which increases code reusability. For example, a single
ArrayList<E>
class can be used to store elements of any type. - Enhanced Performance: By eliminating the need for type casting, generics can lead to more efficient code, reducing the overhead of unnecessary type checking at runtime.
Evaluating Responses:
A comprehensive answer should highlight the key benefits of generics in Java, such as type safety, elimination of casting, code reuse, and improved performance. Candidates should provide examples or scenarios where the use of generics is advantageous over non-generic code.
Q10: How do you synchronize access to an object in a multi-threaded environment in Java?
Question Explanation:
Synchronization in Java is crucial for ensuring that only one thread can access a resource at a time, preventing race conditions and ensuring thread safety. This question tests the candidate’s knowledge of concurrent programming in Java.
Expected Answer:
Java provides several mechanisms for synchronization, including:
- Synchronized Methods: Declaring a method as
synchronized
locks the object for any thread executing this method, ensuring that only one thread can execute any synchronized method of that object at a time. - Synchronized Blocks: Instead of locking the whole method, Java allows synchronization of a block of statements within a method, reducing the scope of synchronization and potentially improving performance.
- Locks in the
java.util.concurrent.locks
package: Java provides a more flexible and sophisticated set of locking mechanisms through theLock
interface and its implementations, such asReentrantLock
. These allow more control over lock acquisition and release. - Volatile Keyword: While not a synchronization technique per se,
volatile
is used to indicate that a variable’s value will be modified by different threads and ensures the visibility of changes to variables across threads.
Evaluating Responses:
Look for detailed explanations of different synchronization techniques, including when and how each should be used. A strong answer will cover synchronized methods and blocks, the use of locks, and the role of the volatile
keyword, along with examples or scenarios illustrating their application.
Q11: Can you differentiate between ==
and .equals()
in Java?
Question Explanation:
In Java, ==
and .equals()
are used to compare objects, but they serve different purposes and work differently. Understanding the distinction is crucial for correctly implementing comparisons in Java applications.
Expected Answer:
==
Operator: The==
operator is used to compare primitives and references. For primitives, it checks if the values are identical. For object references, it checks if both references point to the same object in memory, not comparing the objects themselves..equals()
Method: The.equals()
method is intended for comparing the content of objects. The default implementation of.equals()
in theObject
class checks for reference equality, similar to==
, but it can be overridden in custom classes to compare the objects based on their properties.
For example, two different String
objects containing the same text would not be equal according to ==
(since they are different objects in memory), but .equals()
would return true if it compares the text content of the strings.
Evaluating Responses:
A good response will clearly explain the difference between ==
for checking reference equality and .equals()
for checking object content equality. Examples to illustrate the differences, especially with mutable and immutable objects, will demonstrate a deeper understanding.
Q12: What are lambda expressions, and how do they enhance Java?
Question Explanation:
Lambda expressions were introduced in Java 8 to enable functional programming features. They provide a clear and concise way to represent one method interface using an expression. This question assesses the candidate’s familiarity with functional programming in Java and the benefits of using lambda expressions.
Expected Answer:
Lambda expressions are a feature that allows the creation of anonymous methods (or functions) without a name. They are useful for implementing functional interfaces (interfaces with only one abstract method) in a concise way.
Benefits of lambda expressions in Java include:
- Brevity and Clarity: Lambda expressions are less verbose than traditional anonymous classes, making code more readable and concise.
- Ease of Use with Functional Interfaces: They make it easy to instantiate interfaces for single-method use cases, like event listeners or simple Runnable tasks.
- Enhanced Functional Programming: Lambda expressions support functional programming by enabling operations on collections (such as filter, map, and reduce) to be expressed cleanly and efficiently.
- Parallel Processing: Lambda expressions work well with Java’s Streams API, allowing for efficient parallel processing without the boilerplate code required by traditional loops and anonymous classes.
Evaluating Responses:
Candidates should explain what lambda expressions are, highlighting their syntax and how they can be used to implement functional interfaces. Responses should also discuss the benefits of lambda expressions, particularly in making code more concise, enhancing functional programming, and facilitating operations on collections. Examples illustrating the use of lambda expressions in practical scenarios will add depth to the answer.
Q13: Explain the concept of Java streams and their benefits.
Java streams represent a significant feature introduced in Java 8, enabling functional-style operations on streams of elements. This Java interview question tests the candidate’s understanding of streams and how they can be used to simplify Java code, especially for collections manipulation.
Expected Answer:
Java streams are a sequence of elements supporting sequential and parallel aggregate operations. They facilitate the processing of collections of objects in a functional style, making code more readable and concise.
Benefits of using Java streams include:
- Simplified Collection Processing: Streams provide a high-level abstraction for performing operations on collections, such as filtering, mapping, sorting, and more, using simple method calls.
- Readability and Conciseness: Stream operations are often more readable and concise than traditional loop-based processing, making the code easier to understand and maintain.
- Parallelism: Streams support parallel execution of operations without the need for explicit multithreading code, improving performance on multicore processors.
- Functional Programming Style: Streams facilitate a functional programming style in Java, promoting immutability, statelessness, and side-effect-free functions, which can lead to fewer bugs and cleaner code.
Evaluating Responses:
A comprehensive answer should highlight the key features and benefits of Java streams, such as simplifying collection processing, improving code readability, enabling easy parallelism, and facilitating functional programming. Look for explanations that include examples of stream operations, such as filtering, mapping, and reducing, to demonstrate an understanding of how streams can be applied in practical scenarios.
Q14: How can you manage error handling in Java applications?
Question Explanation:
Error handling is a critical aspect of robust Java application development. This question explores the candidate’s knowledge of Java’s error handling mechanisms and best practices for managing exceptions and errors in Java applications.
Expected Answer:
Java provides a comprehensive framework for error handling, primarily through the use of exceptions. Key practices for managing error handling in Java applications include:
- Try-Catch-Finally Blocks: These are used to catch exceptions that occur in a block of code, with the
finally
block executing regardless of whether an exception was caught. This mechanism is used to catch and handle exceptions explicitly. - Throwing Exceptions: Java allows methods to throw exceptions when an error occurs, passing the responsibility of handling the error to the calling method. This is achieved using the
throw
keyword and declaring exceptions with thethrows
keyword in the method signature. - Custom Exceptions: Developers can define custom exception classes that extend either
Exception
orRuntimeException
, allowing for more descriptive and meaningful exceptions tailored to the application’s needs. - Using Assertions: Assertions can be used to validate assumptions in code, throwing an
AssertionError
if the assumption fails. This is mainly used for debugging purposes. - Best Practices: Include using specific exception types for different errors, documenting exceptions thrown by methods, and avoiding the use of exceptions for flow control.
Evaluating Responses:
Candidates should demonstrate a comprehensive understanding of Java’s error handling mechanisms, including try-catch-finally blocks, throwing and catching exceptions, creating custom exceptions, and using assertions. Look for answers that also mention best practices in exception handling, such as specificity in catching exceptions, proper use of unchecked exceptions, and the importance of clean-up in finally blocks.
Q15: Describe the process and benefits of using annotations in Java.
Question Explanation:
Annotations in Java are a form of metadata that provide data about a program but are not part of the program itself. They have no direct effect on the operation of the code they annotate. This Java interview question gauges the candidate’s understanding of annotations and their role in simplifying Java development.
Expected Answer:
Annotations in Java are used to provide additional information about the program to the compiler, development tools, and runtime environments. They can be used for a wide range of purposes, from compiler instructions to runtime processing.
Benefits of using annotations include:
- Code Simplification: Annotations can replace configuration files, making the codebase cleaner and easier to understand.
- Framework Integration: Many Java frameworks and libraries use annotations extensively for declarative programming. For example, annotations are used in Spring for dependency injection, in JPA for object-relational mapping, and in JUnit for defining test cases.
- Improved Code Analysis: Annotations can be used by tools and IDEs to detect errors, generate code, and enforce coding standards.
- Runtime Processing: Annotations can be accessed at runtime using reflection, allowing for dynamic behavior modifications based on the annotations present.
Evaluating Responses:
Look for explanations that detail the use of annotations in providing metadata about the program to the compiler and runtime, as well as their benefits in simplifying code, integrating with frameworks, and improving code analysis. A strong response might also include examples of common annotations (@Override
, @Deprecated
, @SuppressWarnings
, @Entity
, etc.) and how they are used in Java development.
Java Interview Questions and Answers Conclusion
The above Java interview questions have been carefully curated to cover various aspects of Java programming, from fundamental concepts to advanced features. They aim to not only gauge a candidate’s technical knowledge but also their ability to apply Java principles in real-world scenarios. In an interview setting, these questions can help illuminate a candidate’s problem-solving approach, understanding of Java’s core principles, and proficiency in writing clean, efficient code. Employing such targeted questions is crucial in identifying the right Java engineer who can contribute significantly to your projects and the broader objectives of your organization.