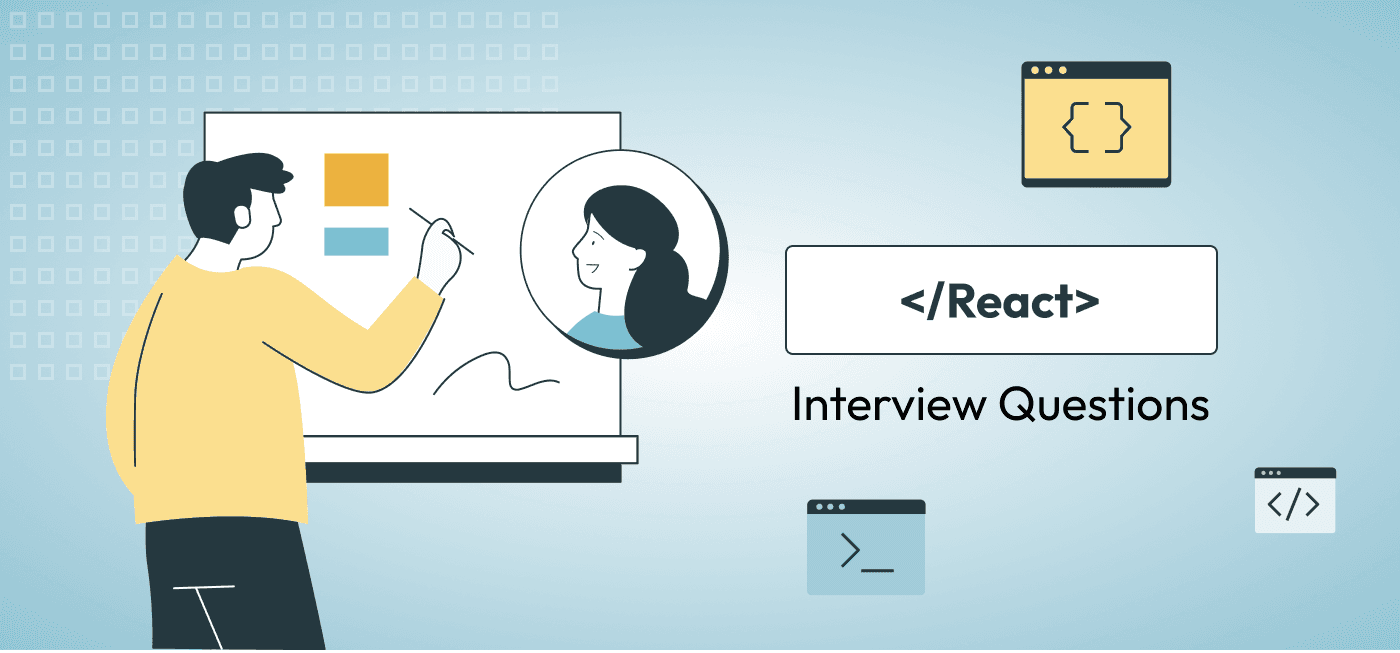
Hiring + recruiting | Blog Post
15 React Interview Questions and Answers for Hiring React Engineers
Todd Adams
Share this post
The quest for excellence in modern web development often leads us to React, a declarative, efficient, and flexible JavaScript library for building user interfaces. In the competitive landscape of software engineering, identifying and hiring adept React engineers is pivotal for the success of projects that demand dynamic and responsive web applications. This post is crafted to aid hiring managers and technical interviewers in assessing candidates’ proficiency in React through great React interview questions and answers. It delves into foundational concepts, best practices, and advanced features of React, ensuring a comprehensive evaluation of a candidate’s capability to tackle real-world challenges.
React Interview Questions
Q1: What is JSX and why is it used in React?
Question Explanation
JSX stands for JavaScript XML. It allows us to write HTML elements in JavaScript and place them in the DOM without any createElement()
and appendChild()
methods. JSX is a syntactic sugar for React’s createElement()
function, making the code more readable and expressive, similar to the template languages used in other frameworks.
Expected Answer
JSX combines the power of JavaScript with the simplicity of HTML. It lets developers write UI components that visually resemble the layout they produce, but with the full power of JavaScript. JSX compiles down to JavaScript, transforming HTML-like text in JavaScript files into React elements.
- Readability: JSX makes components easier to read and write. It visually resembles the layout of the UI, making it more intuitive for developers to understand the structure of the application’s UI.
- React Element Creation: Behind the scenes, JSX is converted into calls to
React.createElement()
. This abstraction improves the development experience and efficiency. - Error Detection: JSX enhances compile-time error checking. If HTML syntax is incorrect, the code won’t compile, helping to catch errors early in the development process.
JSX is not required to use React but is widely adopted due to its simplicity and the productivity boost it provides.
Evaluating Responses
A strong candidate will mention the syntax simplification JSX brings to React development and its role in making the code more readable and maintainable. They might also discuss the transpilation process (e.g., Babel) that converts JSX into JavaScript. Recognizing JSX as syntactic sugar over React.createElement()
shows a deep understanding. Bonus points if the candidate mentions JSX’s contribution to error detection and debugging.
Q2: Can you explain the virtual DOM and its benefits?
Question Explanation
The Virtual DOM is a concept at the heart of React’s high performance. It’s a lightweight copy of the actual DOM in memory. React creates a virtual DOM tree whenever a component renders. Then, using the diffing algorithm, it compares the new virtual DOM with the previous version to determine the minimum number of operations needed to update the real DOM.
Expected Answer
- Performance Improvement: By minimizing direct manipulation of the DOM, which is slow, React improves application performance. The virtual DOM is fast because it’s a lightweight JavaScript object.
- Efficient Updates: The diffing algorithm identifies changes in the virtual DOM and updates only those elements in the real DOM, rather than re-rendering the entire DOM tree. This selective update process significantly reduces the time and computational resources needed.
- Batching: React batches multiple DOM updates into a single update cycle to optimize performance further. This process minimizes the number of reflows and repaints, leading to smoother animations and interactions.
Evaluating Responses
Candidates should recognize the virtual DOM as a performance optimization technique rather than a necessity for building web applications. Understanding that the virtual DOM allows React to be declarative, letting developers write code as if the whole application is rendered on each change, while React takes care of efficiently updating the DOM, is key. High-quality answers may also discuss limitations or misconceptions about the virtual DOM.
Q3: How do you manage state in React applications?
Question Explanation
State management is crucial for controlling the behavior and rendering of React components. It involves tracking changes to variables that determine the output of render functions. React provides built-in hooks like useState
and useReducer
for function components, and class components have their own state management methods.
Expected Answer
- useState Hook: For simple state management in functional components,
useState
provides a way to declare state variables. It returns an array containing the current state value and a function to update it. - useReducer Hook: For more complex state logic that involves multiple sub-values or when the next state depends on the previous one,
useReducer
is more suited. It also makes transitioning to Redux easier if needed for global state management. - Context API: When you need to pass data through the component tree without having to pass props down manually at every level, the Context API is useful. It’s great for storing state that needs to be accessible by many components at different nesting levels.
- External Libraries: For large-scale applications, external libraries like Redux or MobX can offer more powerful solutions for state management, providing capabilities such as centralized state, middleware, and more predictable state updates with actions and reducers.
Evaluating Responses
Candidates should display familiarity with both React’s built-in state management solutions and when to consider external state management libraries. Responses should reflect an understanding of the trade-offs between simplicity and functionality. Knowledge of the Context API’s role in avoiding prop drilling and when it’s appropriate to use external libraries demonstrates depth in managing complex state in React applications.
Q4: Describe the lifecycle of a React component.
Question Explanation
Understanding the lifecycle of a React component is fundamental for effective UI development. The lifecycle encompasses the series of events from the birth to the death of a component. It includes mounting (adding nodes to the DOM), updating (re-rendering due to changes in props or state), and unmounting (removing a component from the DOM).
Expected Answer
- Mounting: The process begins with instantiation and includes methods like
constructor()
,getDerivedStateFromProps()
,render()
, andcomponentDidMount()
. The component is created and inserted into the DOM. - Updating: This phase occurs when a component’s props or state change, triggering re-rendering. Methods involved include
getDerivedStateFromProps()
,shouldComponentUpdate()
,render()
,getSnapshotBeforeUpdate()
, andcomponentDidUpdate()
. - Unmounting: The final phase of the lifecycle, where the component is removed from the DOM. The method
componentWillUnmount()
is called, allowing cleanup of resources, invalidating timers, or canceling network requests.
Evaluating Responses
Candidates should clearly outline the three main phases of a component’s lifecycle and describe the purpose and typical use cases of the key lifecycle methods. A good answer might include recent updates to the lifecycle with React 16.3, such as the introduction of new lifecycle methods (getDerivedStateFromProps
and getSnapshotBeforeUpdate
) and the deprecation of others (componentWillMount
, componentWillReceiveProps
, and componentWillUpdate
). Awareness of hooks like useEffect
for managing side effects in functional components indicates a comprehensive understanding of React’s lifecycle and modern practices.
Q5: What are hooks in React and can you give examples of commonly used hooks?
Question Explanation
Hooks are functions that let you “hook into” React state and lifecycle features from function components. Introduced in React 16.8, hooks allow you to use state and other React features without writing a class. This React interview question assesses the candidate’s familiarity with the concept and use cases of hooks, which are essential for writing modern React applications.
Expected Answer
Hooks in React are designed to allow functional components to manage state and side effects, similar to class components but in a more succinct and straightforward way. The most commonly used hooks include:
- useState: This hook allows you to add state to functional components. For example,
const [count, setCount] = useState(0);
creates acount
state variable, andsetCount
is a method to update its value. - useEffect: It lets you perform side effects in function components, similar to lifecycle methods in class components. It can be used for data fetching, subscriptions, or manually changing the DOM.
- useContext: This hook allows you to access React context without wrapping the component in a Consumer. It’s useful for accessing shared data across many components.
- useReducer: Use it for managing state logic that involves multiple sub-values or when the next state depends on the previous one. It’s an alternative to
useState
that’s more suited for complex state logic. - useRef: This hook can access and interact with DOM elements directly. It’s used to store a mutable reference to a DOM element.
Evaluating Responses
Candidates should demonstrate an understanding of how hooks simplify the management of state and lifecycle events in functional components. They should provide examples of common hooks and their use cases. Awareness of hooks represents a grasp of modern React development practices. Mentioning custom hooks as a means to extract component logic into reusable functions would indicate an advanced understanding.
Q6: How do you handle forms in React?
Question Explanation
Form handling in React involves understanding how to collect input from the user, validate it, and submit it. React provides two approaches: controlled components, where form data is handled by the React component’s state, and uncontrolled components, which use refs to access the form data directly from the DOM.
Expected Answer
In React, forms are typically handled by using controlled components. This approach involves setting the value of form elements via state and updating that value based on user input. For example:
const [value, setValue] = useState("");
const handleChange = (event) => {
setValue(event.target.value);
};
<form onSubmit={handleSubmit}>
<input type="text" value={value} onChange={handleChange} />
<button type="submit">Submit</button>
</form>
For validation, you can add checks directly in the onChange
handler or use a form validation library like Formik or React Hook Form, which simplify the process.
Uncontrolled components, on the other hand, use useRef
to directly access form elements. This approach is less common but may be preferred for certain use cases where you want to avoid re-rendering on every input change.
Evaluating Responses
Candidates should show an understanding of controlled and uncontrolled components in React and the scenarios where one might be preferred over the other. A good answer will include examples of how to implement form handling, possibly including validation. Awareness of libraries that aid in form handling and validation shows a practical approach to development.
Q7: Explain the concept of props in React and how they differ from state.
Question Explanation
Props (short for “properties”) and state are both plain JavaScript objects in React. They hold information that influences the output of render, but they are used differently within components. Understanding the distinction and appropriate use of props and state is fundamental to building React applications.
Expected Answer
- Props: Props are read-only and are used to pass data from a parent component to a child component. They are not modifiable within the child component; any change to prop values should occur in the parent component. Props are used for component configuration and are the mechanism for passing data down the component tree.
- State: Unlike props, state is local and mutable. It is used to hold data that changes over time and affects the component’s rendering. Changes to state can trigger re-renders of the component and its children. State is managed within the component (similar to variables declared within a function).
The main difference is in the ownership and mutability: props are passed to a component (similar to function parameters) and are immutable by the component that receives them, whereas state is managed within the component and can be changed by the component itself.
Evaluating Responses
Candidates should clearly articulate the distinction between props and state, including their mutability and typical use cases. Understanding that props are immutable within the receiving component and are used for configuring components, while state is mutable and used for data that changes over time, is crucial. Mentioning when to lift state up for shared state management between components indicates a deeper understanding of React’s data flow.
Q8: What are higher-order components (HOCs) and when would you use them?
Question Explanation
Higher-order components (HOCs) are an advanced pattern in React for reusing component logic. They are functions that take a component and return a new component, allowing you to abstract and reuse code. This React interview question help assess when and how to use HOCs is key to structuring and organizing large React applications effectively.
Expected Answer
A Higher-Order Component (HOC) is a function that takes a component and returns a new component with additional props or functionality. It’s a pattern used to share common functionality between components without repeating code. For example, an HOC can be used to add state management or data fetching capabilities to a component.
function withSubscription(WrappedComponent, selectData) {
return class extends React.Component {
constructor(props) {
super(props);
this.state = {
data: selectData(DataSource, props),
};
}
componentDidMount() {
// Subscribe or perform data fetching here
}
componentWillUnmount() {
// Clean up
}
render() {
return <WrappedComponent data={this.state.data} {...this.props} />;
}
};
}
HOCs are useful in many scenarios, such as handling user authentication, data fetching, and applying styles. They promote code reusability and composability in React applications.
Evaluating Responses
The candidate should demonstrate an understanding of the HOC pattern, including its syntax and use cases. A strong answer includes examples of how HOCs can abstract and reuse code across components. Additionally, awareness of the potential downsides of HOCs, such as prop collisions and the complexity they can introduce, indicates a nuanced understanding of their use in application design.
Q9: Can you explain how context works in React and provide a use case?
Question Explanation
The React Context API allows for data to be shared globally across a React application, sidestepping the need to pass props down manually through every level of the component tree. This question probes the candidate’s understanding of Context as a tool for state management and its appropriate application scenarios.
Expected Answer
Context in React provides a way to pass data through the component tree without having to pass props down manually at every level. It’s used to share values like themes, user information, or UI states that are needed by many components within an application.
To use Context, you’ll typically define a new Context object using React.createContext()
, then use a Provider
to pass the current context value down the tree. Any component that needs to access this value can use the Consumer
component or the useContext
hook.
A common use case for Context is theming. By defining a ThemeContext
, you can easily toggle between light and dark modes across your entire application without prop drilling:
const ThemeContext = React.createContext();
function ThemeProvider({ children }) {
const [theme, setTheme] = useState("light");
// Toggle between "light" and "dark"
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === "light" ? "dark" : "light"));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
Components anywhere in the application can access the theme and toggle it without needing to receive it directly as a prop.
Evaluating Responses
Candidates should demonstrate an understanding of when and why to use Context, highlighting its role in avoiding prop drilling and simplifying state management across multiple components. Describing how to set up a Context and use it within an application, as well as potential pitfalls like unnecessary re-renders or overuse, indicates depth in understanding React’s Context API.
Q10: How does React implement reusability in components?
Question Explanation
Component reusability is a core concept in React, allowing developers to create flexible, efficient, and maintainable code. This question assesses the candidate’s knowledge of patterns and practices for reusing components, which is key to building scalable React applications.
Expected Answer
React promotes reusability through its component-based architecture, enabling developers to create encapsulated components that manage their own state and can be reused throughout the application. There are several ways to implement reusability in React:
- Composition: Utilizing component composition, where components are used as children or as parts of other components, allows for flexible reusability. It’s the preferred method for reusing component logic and UI in React.
- Higher-Order Components (HOCs): As discussed earlier, HOCs are functions that take a component and return a new component, enhancing the original with additional properties or methods. This pattern is useful for sharing common functionality across components.
- Custom Hooks: Custom hooks allow you to extract component logic into reusable functions. By encapsulating logic in hooks, you can share behaviors across multiple components without changing their hierarchy.
- Render Props: The render prop pattern involves a component receiving a function that returns a React element and calls it instead of implementing its own render logic. This pattern is useful for sharing code between components with a flexible and reusable API.
Evaluating Responses
The candidate’s response should encompass various methods for achieving reusability in React, including composition, higher-order components, custom hooks, and the render props pattern. An understanding of how these patterns and practices enable efficient and maintainable code development, along with examples, indicates a solid grasp of React’s component-based architecture and reusability strategies.
Q11: What is React Fiber and how does it enhance React?
Question Explanation
React Fiber is a complete reimplementation of React’s core algorithm, introduced in React 16. It aims to improve the responsiveness and performance of complex applications by enabling incremental rendering of the component tree. Understanding Fiber is crucial for grasping the underpinnings of React’s advanced features.
Expected Answer
React Fiber is the new reconciliation engine or the core algorithm behind React 16 and later versions. It enhances React by enabling incremental rendering—breaking the rendering work into chunks and spreading it out over multiple frames to avoid blocking the browser’s main thread.
Key benefits include:
- Improved Performance: By splitting rendering tasks into small, manageable units, Fiber ensures that the main thread is not blocked, leading to smoother UI interactions and animations.
- Better User Experience: Fiber’s ability to prioritize updates based on their importance (such as prioritizing user interactions over background data syncing) improves the perceived performance of web applications.
- Concurrency: Fiber lays the groundwork for concurrent mode, which allows React apps to prepare multiple versions of the UI at the same time. This enables React to interrupt a low-priority rendering process to attend to a higher-priority update if needed.
Evaluating Responses
An ideal answer will mention Fiber’s role in enabling incremental rendering and its impact on application performance and user experience. Candidates should also recognize the introduction of Fiber as a foundational update that supports future React features, such as concurrent mode. Demonstrating knowledge of how Fiber operates under the hood—though not required for most development work—indicates a deep understanding of React’s core principles.
Q12: How would you approach error handling in React components?
Question Explanation
Effective error handling is critical for maintaining a good user experience and application stability. React provides mechanisms to gracefully catch and handle errors in components. This question evaluates the candidate’s ability to implement robust error handling within a React application.
Expected Answer
In React, error boundaries are the primary means for error handling in components. An error boundary is a component that catches JavaScript errors anywhere in its child component tree, logs those errors, and displays a fallback UI instead of the component tree that crashed.
Error boundaries are implemented using two lifecycle methods:
static getDerivedStateFromError(error)
: This lifecycle method is used to render a fallback UI after an error has been thrown. It receives the error that was thrown as a parameter and returns a new state.componentDidCatch(error, info)
: This method is used to log error information. It catches errors in any components below and logs them.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can also log the error to an error reporting service
logErrorToMyService(error, errorInfo);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
To use it, simply wrap any component with an ErrorBoundary
:
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
Evaluating Responses
Candidates should mention the use of error boundaries for catching and handling errors in React components. A well-rounded answer will include an explanation of how to implement an error boundary and scenarios where it might be particularly useful. Awareness of limitations, such as not catching errors in event handlers and asynchronous code, indicates a thorough understanding of error handling in React.
Q13: Discuss the differences between controlled and uncontrolled components in React.
Question Explanation
Understanding the difference between controlled and uncontrolled components is essential for managing form elements in React. This React interview question and concept highlights React’s flexibility in handling user input and form submission, illustrating two distinct approaches to data flow within components.
Expected Answer
- Controlled Components: In a controlled component, form data is handled by the React component’s state. The input form element’s value is controlled by React, meaning that every state mutation has an associated handler function. This approach makes it easy to validate or manipulate user input. For example, using the
useState
hook to manage the input’s state and attaching anonChange
event handler to update this state.
const [value, setValue] = useState("");
const handleChange = event => {
setValue(event.target.value);
};
<input type="text" value={value} onChange={handleChange} />
- Uncontrolled Components: Uncontrolled components, on the other hand, store their own state internally and query the DOM using a ref to find the current value when it’s needed. This is more akin to traditional HTML.
const inputRef = useRef();
const handleSubmit = event => {
alert('A name was submitted: ' + inputRef.current.value);
event.preventDefault();
};
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" ref={inputRef} />
</label>
<button type="submit">Submit</button>
</form>
Evaluating Responses
Candidates should clearly describe both controlled and uncontrolled components, including how they manage state and interact with form data. A good response will include examples of each and might discuss the pros and cons, such as the more straightforward implementation of uncontrolled components versus the greater flexibility and scalability of controlled components. Understanding when to use each approach based on the application’s needs is key.
Q14: What are React Portals and where can they be beneficial?
Question Explanation
React Portals provide a first-class way to render children into a DOM node that exists outside the DOM hierarchy of the parent component. This concept is crucial for managing modals, pop-ups, and tooltips, especially when dealing with CSS stacking context and accessibility.
Expected Answer
React Portals allow for child components to be rendered into a DOM node that exists outside the usual parent-child hierarchy, essentially enabling a child to break out of its DOM tree. This is particularly useful for UI elements that need to visually ‘break out’ of their parent container, such as modals, dropdowns, or tooltips.
To create a portal, you can use ReactDOM.createPortal(child, container)
:
const modalRoot = document.getElementById('modal-root');
class Modal extends React.Component {
render() {
return ReactDOM.createPortal(
this.props.children,
modalRoot
);
}
}
This method takes two arguments: the JSX of the child and the DOM element to insert the child into. Despite the child being rendered elsewhere in the DOM, it remains part of the React data flow and can access context like any regular component.
Evaluating Responses
Candidates should understand the purpose of React Portals and how they’re used to render components outside the parent component’s DOM hierarchy. Examples involving modals, tooltips, or other floating elements demonstrate practical knowledge. Discussing scenarios where portals are beneficial, such as avoiding z-index issues or when dealing with overflow hidden problems, indicates a comprehensive understanding of this feature.
Q15: How do you optimize performance in a React application?
Question Explanation
Performance optimization is crucial for maintaining a smooth user experience in React applications. This involves understanding React’s rendering behavior and knowing the tools and techniques to prevent unnecessary re-renders and optimize component updates.
Expected Answer
Performance optimization in React can be approached from several angles:
- Using
React.memo
for Functional Components: Wraps a component and prevents it from re-rendering if its props haven’t changed, similar toPureComponent
for class components. shouldComponentUpdate
in Class Components: Allows you to prevent unnecessary re-renders by comparing current and next props and state, returningfalse
if an update isn’t needed.- Code Splitting with
React.lazy
andSuspense
: Allows components to be loaded only when needed, reducing the initial load time of the app. - Optimizing Context: By splitting contexts into multiple providers based on their update frequency to avoid unnecessary re-renders of consumers that do not care about certain updates.
- Using Keys in Lists: Helps React identify which items have changed, are added, or are removed, improving performance in list rendering.
- Avoiding Inline Functions and Objects in JSX: Inline functions and objects are recreated on every render, potentially leading to unnecessary re-renders.
- Memoizing Expensive Computations: Using
useMemo
to store the results of expensive function calls so that you don’t need to recompute them on every render.
Evaluating Responses
An ideal answer will cover various techniques for optimizing performance, including both React-specific features (like React.memo
and shouldComponentUpdate
) and general best practices (such as code splitting and memoization). Knowledge of profiling and debugging performance issues using React Developer Tools indicates a candidate’s ability to diagnose and address performance bottlenecks effectively.
React Interview Questions and Answers Conclusion
These React interview questions are designed to unearth the depth of a candidate’s understanding and practical application of React. They span from foundational knowledge to complex problem-solving scenarios, offering a holistic view of a candidate’s capabilities. In the rapidly evolving domain of web development, proficiency in React is a significant asset. These questions, therefore, are not just inquiries but tools to gauge potential, critical thinking, and the ability to adapt and innovate. The ability of a React engineer to navigate these questions with confidence and clarity is indicative of their readiness to contribute effectively to development projects that require cutting-edge front-end solutions.